Playing keyframe animations
Use the Animation Player to play and control the playback of keyframe animations.
Playing keyframe animations
To play a keyframe animation:
- Create a keyframe animation and a node you want to animate with that animation. See Creating keyframe animations.
- In the Project select the node you want to animate, in the Node Components > Animation section click + Add Animation and select Animation Player.
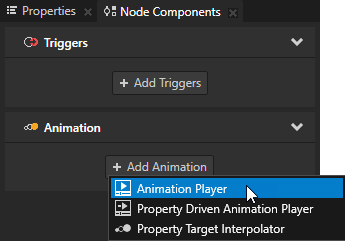
- In the Node Components > Animation section in the Animation Player you created set the Target Animation Timeline property to the animation you want use.
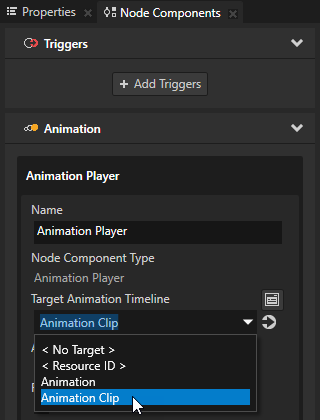
- (Optional) In the Animation Player set the animation playback:
- Autoplay Enabled sets whether the animation starts immediately after Kanzi attaches the node with the Animation Player to the scene graph.
- Relative Playback sets whether the property values set in the animation are added to the property values in the target node or replace the values of these properties.
- Restore Original Values After Playback sets whether the values of the animated properties return to their initial values when the animation ends.
For example, if the initial value of a property you animate is 0 and at the end of the animation the value is 1, when you enable the Restore Original Values After Playback property, Kanzi returns the value of the animated property to 0 when the animation ends. - Playback Mode, Duration Scale, and Repeat Count set in which direction, at what speed, and how many times the animation plays. To make it easier to reuse the animation, it is recommended to set the values of these properties in the Start Animation Playback action instead of the Animation Player. See Controlling keyframe animations.
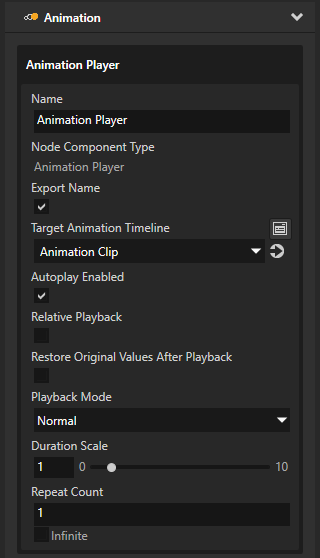
Controlling keyframe animations
You can control keyframe animations using the animation control actions in any trigger.
To control keyframe animations:
- Create a keyframe animation, a node you want to animate with that animation, and add the Animation Player to the node you want to animate. See Playing keyframe animations.
- Create a node you want to use to control a keyframe animation in the node you created in the previous step.
For example, create a Button node.
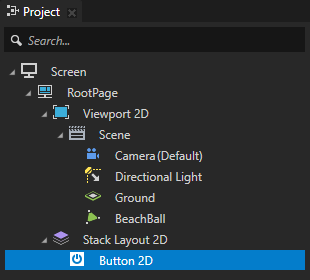
- In the Node Components > Triggers section add the trigger you want to use to control the keyframe animation.
For example, use the Button: Click trigger.
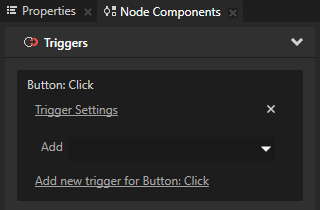
- In the Node Components > Triggers section in the trigger you want to use to control the keyframe animation click the dropdown menu and select an animation control action:
- Start Animation Playback to start an animation
- Pause Animation Playback to pause a running animation
- Resume Animation Playback to resume a paused animation
- Stop Animation Playback to stop a running or paused animation
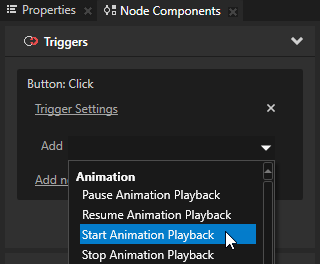
- In the action settings window set the Target Item property to the node that contains the Animation Player you want to control with this action.
If the node contains more than one Animation Player, set the Target Node Component Name property to the name of the Animation Player you want to control with this action.
To control all the Animation Player components in the node, leave the Target Node Component Name property empty.
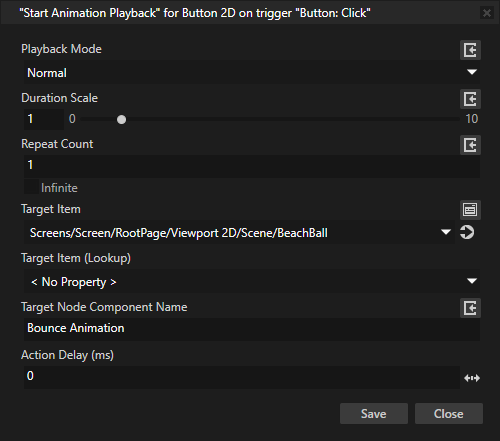
- Set the animation playback:
- Playback Mode property sets how Kanzi plays the animation:
- Normal plays the animation as it is defined in the animation.
- Ping pong first plays the animation as it is defined in the animation and then plays the animation in reverse.
- Reverse plays the animation defined in the animation in reverse.
- Duration Scale property defines the duration of the animation.
For example:- When set to 1, the animation is of the same length as it is defined in the animation.
- When set to 0,5, the animation is half as long as it is defined in the animation.
- When set to 2, the animation is twice as long as it is defined in the animation.
- Repeat Count sets how many times the animation is played. In the Repeat Count property when you enable the Infinite property, the Animation Player plays the animation infinite amount of times.
Kanzi applies the values of the Playback Mode, Duration Scale, and Repeat Count properties in the Start Animation Playback action on top of the values set in the Animation Player.
For example, if in the Animation Player the Playback Mode property is set to Reverse and the Repeat Count property is set to 2, and in the Start Animation Playback action the Playback Mode property is set to Ping pong, the Animation Player plays the animation timeline first twice in reverse and then twice in normal mode.
Getting information about the animation state
When Animation Player starts, stops, or reaches the end of the animation it plays, it sends messages you can intercept to find out about the state of that animation. For example, you can use these messages to chain animations.
To get information about the animation state:
- Create a keyframe animation, a node you want to animate with that animation, and add the Animation Player to the node you want to animate. See Playing keyframe animations.
- In the Project select a node where you want to get the information about an animation played by the Animation Player.
For example, if you use the Animation Player in the BeachBall node, but want to get the information about that animation in the RootPage node, select the RootPage node.
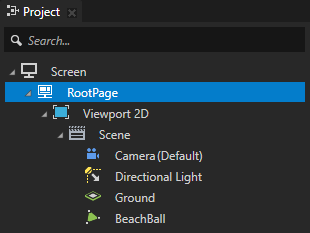
- In the Node Components > Triggers section right-click, select Add Trigger > Animation, and select the trigger which provides the information about the state of animation you need:
- Animation Playback Started trigger intercepts the message an Animation Player sends when it starts the playback of an animation.
- Animation Playback Stopped trigger intercepts the message an Animation Player sends when it stops the playback of an animation.
- Animation Playback Completed trigger intercepts the message an Animation Player sends when it plays an animation to the end.
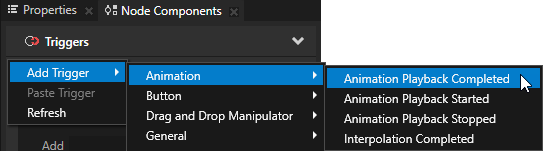
- In the Node Components > Triggers section click Trigger Settings for the trigger you added in the previous step and set the Message Source property to the node which has the Animation Player for which you want to find out the state of the animation it plays.
For example, if you want to get the information about the animation of the BeachBall node in the RootPage node, in the Trigger Settings set the Message Source to the BeachBall node.
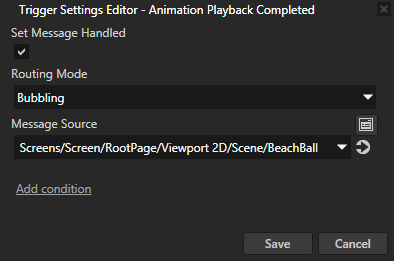
- In the Node Components > Triggers add to the trigger the action you want to use when the Animation Player sends the message your trigger intercepts.
For example, add the Start Animation Playback action and set it to start another animation.
Using Animation Player in the API
To create animation player and set timeline:
// Create animation player.
AnimationPlayerSharedPtr animationPlayer = AnimationPlayer::create(domain, "player");
// Set timeline.
animationPlayer->setTimeline(timeline);
To attach animation player to the node:
node->addNodeComponent(animationPlayer);
To start animation player:
// Start playback in animation player by sending PlayMessage
// to the node where animation player is attached.
AnimationPlayer::PlayMessageArguments playMessageArguments;
playMessageArguments.setPlaybackMode(Timeline::DirectionBehaviorReverse);
playMessageArguments.setDurationScale(2.0f);
playMessageArguments.setRepeatCount(2);
node->dispatchMessage(AnimationPlayer::PlayMessage, playMessageArguments);
To pause animation player:
// Pause playback in animation player by sending PauseMessage
// to the node where animation player is attached.
AnimationPlayer::PauseMessageArguments pauseMessageArguments;
node->dispatchMessage(AnimationPlayer::PauseMessage, pauseMessageArguments);
To resume animation player:
// Resume playback in animation player by sending ResumeMessage
// to the node where animation player is attached.
AnimationPlayer::ResumeMessageArguments resumeMessageArguments;
node->dispatchMessage(AnimationPlayer::ResumeMessage, resumeMessageArguments);
To stop animation player:
// Stop playback in animation player by sending StopMessage
// to the node where animation player is attached.
AnimationPlayer::StopMessageArguments stopMessageArguments;
node->dispatchMessage(AnimationPlayer::StopMessage, stopMessageArguments);
To detach animation player from the node:
node->removeNodeComponent(*animationPlayer);
For details, see the AnimationPlayer
class in the API reference.
See also
Changing the interpolation mode between keyframes
Editing animation clips
Editing timeline sequences
Creating keyframe animations
Creating animations and timelines using the Kanzi Engine API
Animations
Open topic with navigation